In Laravel, it is a common mistake to use PHP’s empty() function to check if a collection is empty. When empty() is used on an empty array [] the boolean value of true is returned. However, using empty() on a collection can cause unexpected results because when a collection has no values the empty() function will return false.
The below screenshot shows an empty array being assigned to the variable $arr1. The screenshot then shows the expected result of true being returned from using var_dump() to display the data type and value of the result of empty($arr1). The screenshot then shows how the empty() function could be used in code with a simple ternary condition that prints ’empty array’.
The screenshot then goes on to illustrate how using empty() on a Laravel collection causes incorrect results. The screenshot shows a collection with no values being assigned to the $collection1 variable. The screenshot then shows the result of var_dump of empty($collection1) is false. The screenshot then shows the empty() function used in code with a simple ternary condition that prints ‘Not empty collection’ even though the collection is empty.
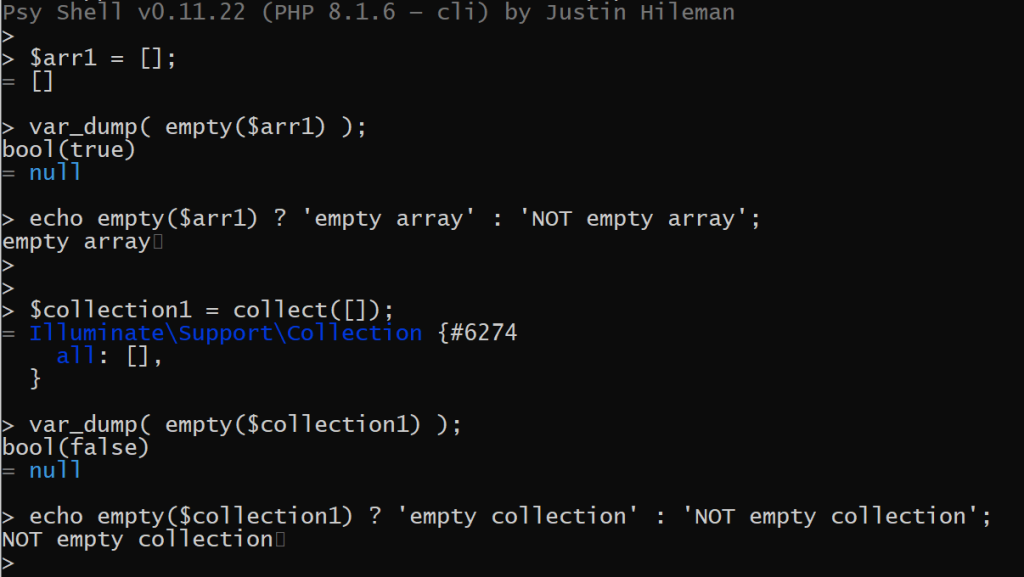
The reason that empty() returns false on a collection is because a collection is an instance of the Illuminat\Support\Collection class. An instance of a class is not considered to be empty in PHP as illustrated by the below screenshot.
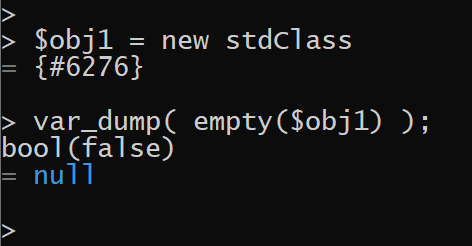
Ways To Check If A Collection Is Empty
Some ways to return correct results when checking if a collection is empty are
- isEmpty() method
- isNotEmpty() method
- count() method
isEmpty() method
The first way to check if a collection is empty is by using the isEmpty() method. This collection method is the most similar to PHP’s empty() function.
This is the explanation of isEmpty() from Laravel’s documentation: The isEmpty
method returns true
if the collection is empty; otherwise, false
is returned
The below screenshot shows how isEmpty() works:
- An empty collection being assigned to the $collection1 variable.
- var_dump is used to display that true is returned when the isEmpty() method is called on an empty collection.
- A ternary condition is used to display how calling isEmpty() on a collection can be used in code to check if the collection is empty.
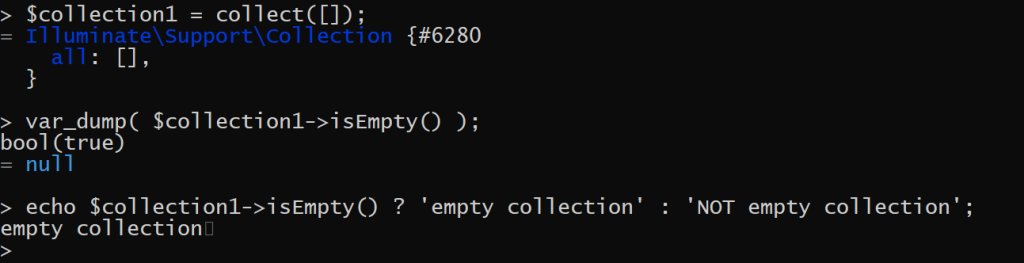
isNotEmpty() method
The second way to check if a collection is empty is by using the isNotEmpty() method.
This is the explanation of isNotEmpty() from Laravel’s documentation: The isNotEmpty
method returns true
if the collection is not empty; otherwise, false
is returned
The below screenshot shows how isNotEmpty() works:
- An empty collection being assigned to the $collection1 variable.
- var_dump is used to display that false is returned when the isNotEmpty() method is called on an empty collection.
- A ternary condition is used to display how calling isNotEmpty() on a collection can be used in code to check if the collection is not empty.
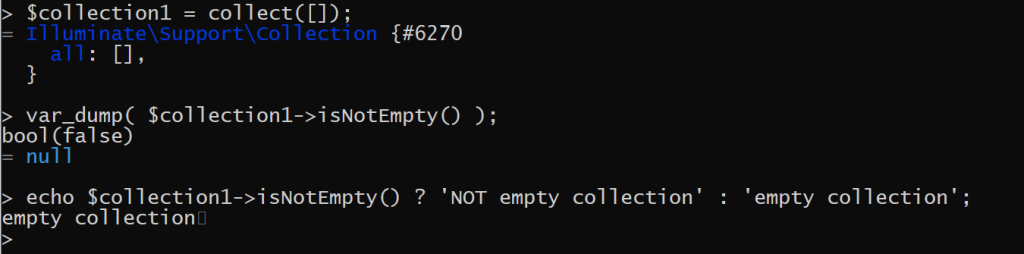
count() method
A third way to check if a collection is empty is by using the count() method.
This is the explanation of count() from Laravel’s documentation: The count
method returns the total number of items in the collection
The below screenshot shows how count() works:
- An empty collection being assigned to the $collection1 variable.
- var_dump is used to display that an int of 0 is returned when the count() method is called on an empty collection.
- A ternary condition is used to display how calling count() on a collection can be used in code to check if the collection is empty.
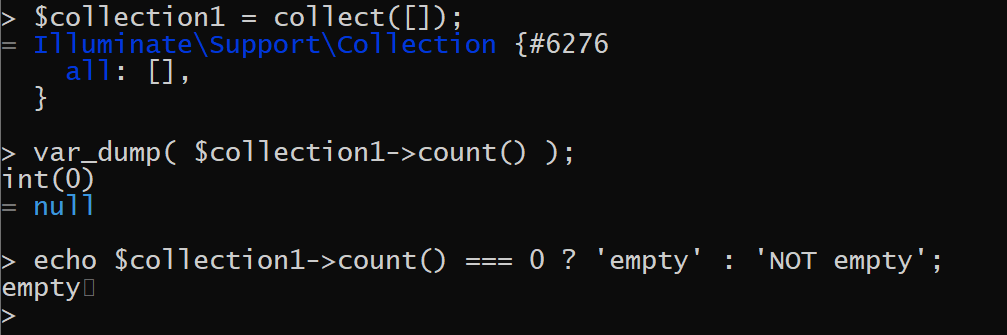
Conclusion
Using PHP’s empty() function to check if a Laravel collection can provide incorrect results. To check if a Laravel collection is empty, use one of the collection methods available such as isEmpty, isNotEmpty, or count.
References:
https://laravel.com/docs/10.x/collections
https://laravel.com/docs/10.x/collections#method-isempty
https://laravel.com/docs/10.x/collections#method-isnotempty
https://laravel.com/docs/10.x/collections#method-count
https://stackoverflow.com/questions/41269198/how-to-check-if-a-laravel-collection-is-empty
https://www.youtube.com/watch?v=vCZ2qsxm8JE