When debugging javascript it is common to use console.log() to print variables or messages to the browser’s dev tools console. The dir() method is another tool for logging that can be useful in certain situations.
From the MDN Web Docs, console.dir() is given the following description: “The method console.dir()
displays an interactive list of the properties of the specified JavaScript object.“
From this description, we can test the differences between console.log() and console.dir() by printing a simple literal object.
let person = { name: "Michael Jordan", age: 58, job: "Retired Basketball Player" } console.log(person); console.dir(person);
Below is a screenshot of the output in Google Chrome’s console.
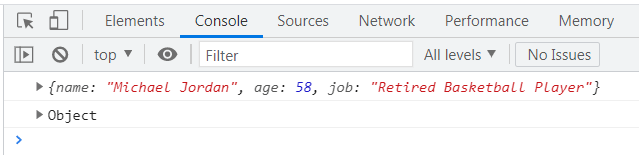
Below is a screenshot of the output with the console messages expanded.
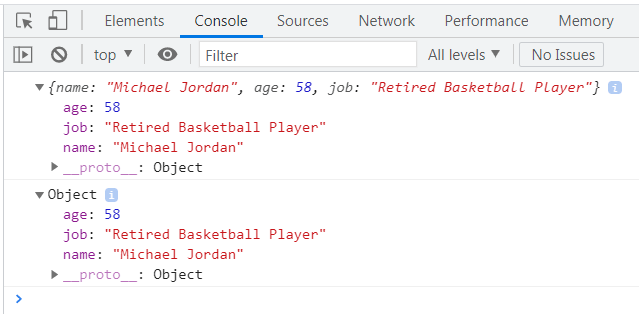
From the above output screenshots, we can see that when console.log() is used many of the properties and values of the object can be viewed. When console.dir() is used just Object is printed to the console. When the console messages are expanded the console.log() and console.dir() methods display the object in the same way with the properties and values being listed.
Many developers try this test and see that console.log() is more helpful when the messages are not expanded and console.log() and console.dir() are basically the same when the messages are expanded. It is then common for developers to decide that console.dir() is not useful and forget that it exist.
However, there are two specific objects that console.dir() can provide helpful information:
- HTML DOM Elements
- Functions
HTML DOM Elements
The below code assigns an HTML input tag to a variable and then logs that HTML DOM element to the console using console.log() and console.dir().
<!DOCTYPE html> <html> <head> </head> <body> <input type="text" id="testInput" name="testInput" value="Hello World!"> <script> let inputEl = document.getElementById('testInput'); console.log(inputEl); console.dir(inputEl); </script> </body> </html>
Below are screenshots of the output. The first screenshot shows that the output of the HTML element from console.log() shows the HTML tag. The output from console.dir() shows as input#testInput and when it is expanded it shows properties and their values for this HTML DOM element. There are too many DOM element properties to show in screenshots so the next two screenshots show some more of the properties.
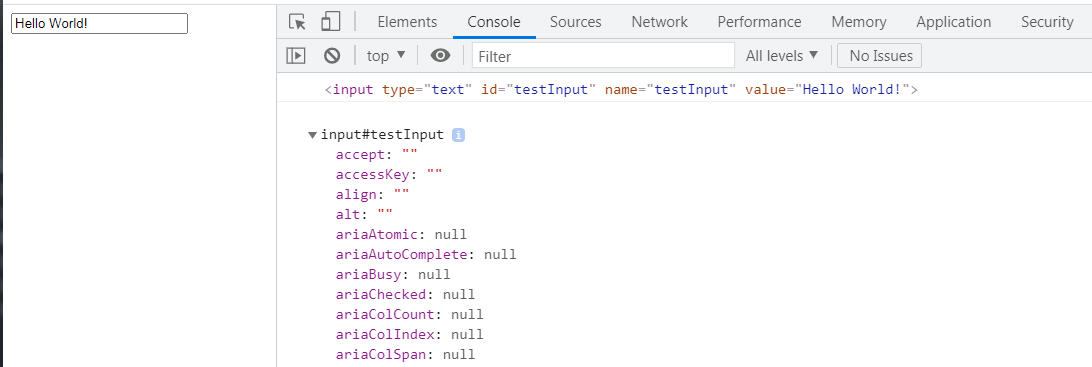
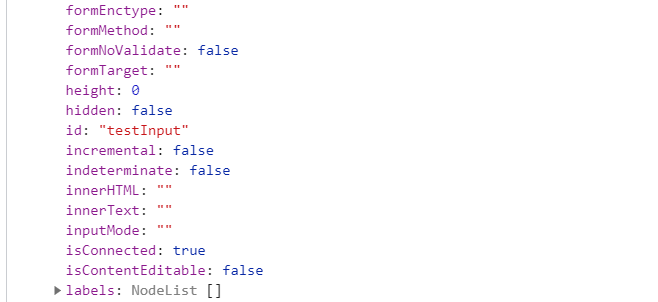
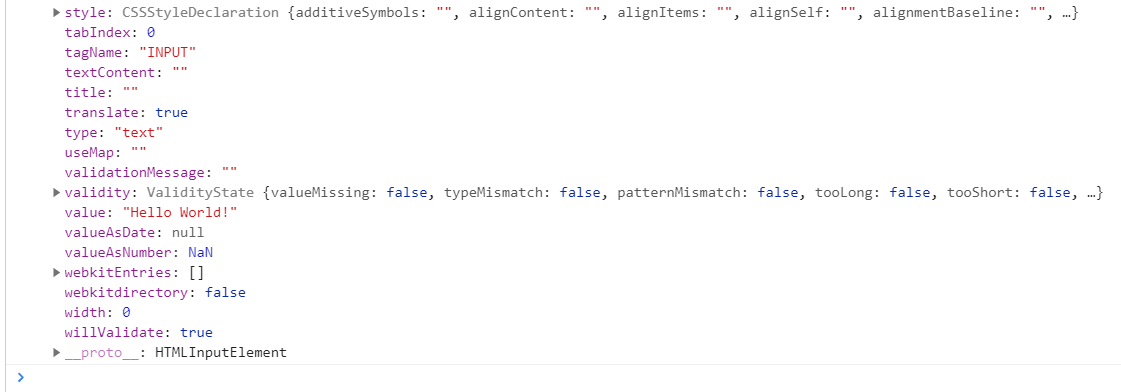
Logging an HTML element with console.dir() can be helpful if you need to view what properties are available to an HTML element and what values are assigned to the properties. Some common useful HTML DOM element properties are value, innerHTML, disabled, parentElement, childNodes, and style. Some of the properties can be expanded like the style property that shows the CSS rules for the HTML element.
Functions
Logging a function with console.log() will print the function or a portion of it. Logging a function with console.dir() will provide more information about the function.
The below code invokes a function and prints the function to the console using console.log() and console.dir().
const sayHello = function() { console.log(sayHello); console.dir(sayHello); console.log("Hello World!"); }; sayHello();
Below is a screenshot of the output of the above code. The console.log() prints f () to show that it is a function and then prints the text of the body of the function. When the function is printed using console.dir() more details about the function is displayed. This is the same information that is displayed when using the debugger in a function.
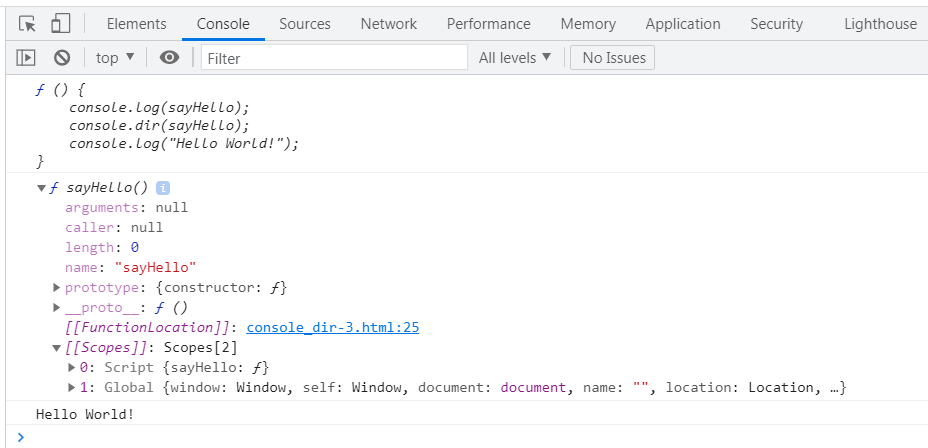
Some of the potential useful function information logged by console.dir() is:
- prototype: The prototype chain is listed.
- [[FunctionLocation]]: This shows what file the function is in and the line number location within that file. Clicking the link will take you directly to the function in the dev tools console where you can review the code or set breakpoints. This can be helpful if you are working in a project with many large files.
- [[Scopes]]: This shows the scopes available to the function. This can be helpful to see what variables and scopes are accessible to the function. This can be helpful debugging nested functions and closure.
The code below shows a closure in [[Scopes]] when a function is logged with console.dir().
function outerFn(){ let i = 0; return function(){ i++; return "invoked " + i + " times"; } } let innerFn = outerFn(); innerFn(); innerFn(); console.dir(innerFn);
In the below console output, the closure of innerFn is highlighted in the red rectangle.
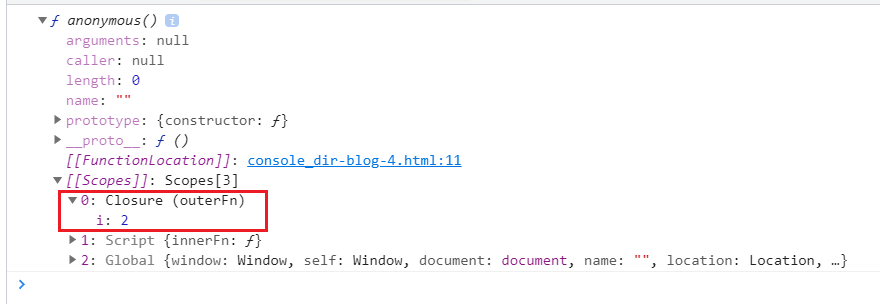
Conclusion
In most cases there is little or no difference in the output of console.log() or console.dir(). However, logging HTML DOM elements or functions with console.dir() provides a listing of their properties and values that can potentially be useful when debugging or learning javascript.
Here is another article you may be interested in: Javascript – How To Add CSS Styles To console.log Messages
References:
https://developer.mozilla.org/en-US/docs/Web/API/Console/dir
https://vitamindev.com/javascript/console-log-vs-console-dir/
https://css-tricks.com/a-guide-to-console-commands/
https://stackoverflow.com/questions/35223591/get-list-of-closure-variables-in-javascript